Expo iOS and Apple Sign In
Docs:
Git commit: This commit contains the changes in this section:
1. Install dependencies
Install Expo Apple Authentication: npx expo install expo-apple-authentication
Install Async Storage: npx expo install @react-native-async-storage/async-storage
TIP
We will need to use Async Storage
to store the the user info, because the Apple Sign In
doesn't return the user info in the callback.
2. Setup iOS project
To enable the Sign In with Apple capability in your app, set the ios.usesAppleSignIn
property to true in your project's app config.
Also need to add the plugin.
// app.json
{
// ...
"expo": {
// ...
"ios": {
// ...
"usesAppleSignIn": true
},
// ...
"plugins": [
// ...
"expo-apple-authentication"
]
}
}
3. Build the app and run on iOS
Then run the following to generate the native project directories for iOS:
npx expo prebuild --platform ios
npx expo run:ios
4. Apple Sign In component
Add the component to App.tsx
:
// App.tsx
...
{Platform.OS === 'ios' && <AppleSignIn />}
...
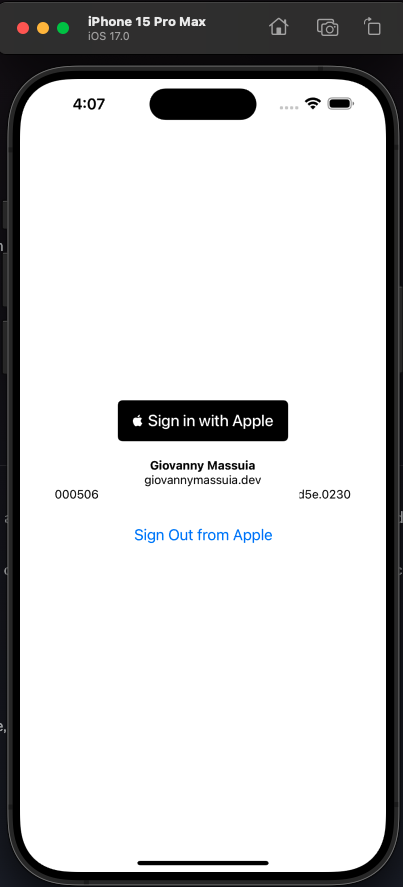
Points of attention for Apple Sign In and iOS
TIP
The name and last of a user is only available in the first sign in, after that it will be null
. Also, this image to create an accoutn
will also only show in the first sign in.
screenshot
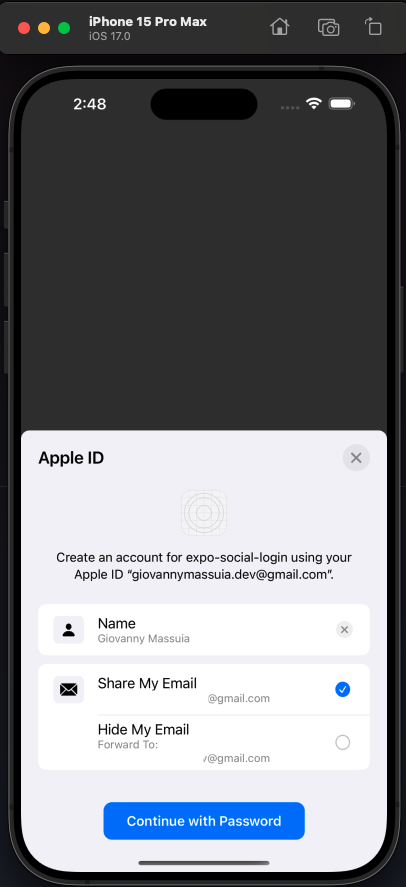
The full name is only shown in the first sign in, after that it will be null
. Make sure you have the fullName
stuff from the response
object.
"fullName": {
"middleName": null,
"nameSuffix": null,
"givenName": "Giovanny",
"familyName": "Massuia",
"nickname": null,
"namePrefix": null
},
TIP
Make sure you have in xcode the following: Sign In with Apple
added to the Signing & Capabilities
tab.
screenshot
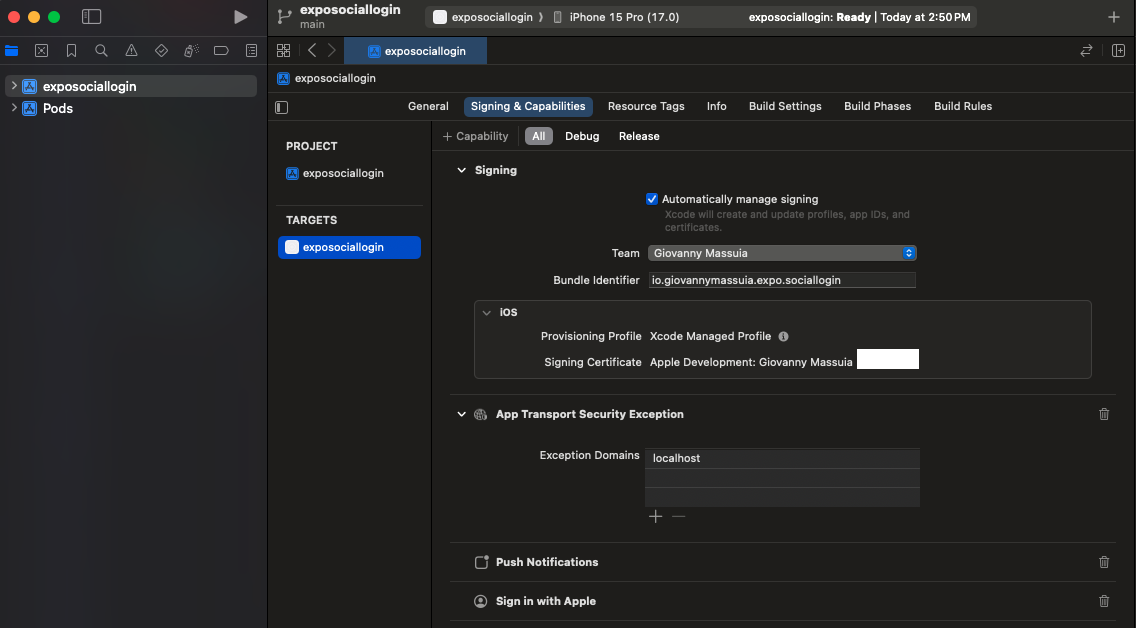